Aula 83 – Loja Online – Adicionando Campo Obrigatório
Aula 83 – Loja Online – Adicionando Campo Obrigatório
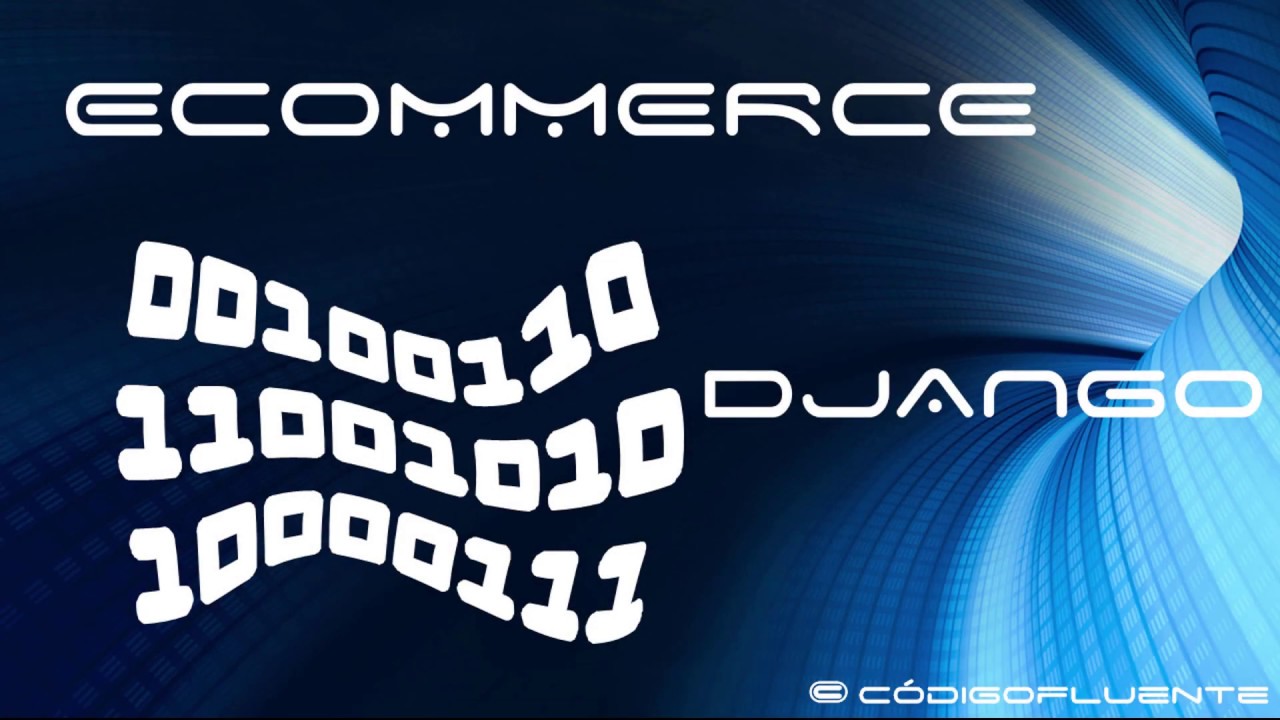
Loja Online – Django
Voltar para página principal do blog
Todas as aulas desse curso
Aula 82 Aula 84
Redes Sociais:
Meus links de afiliados:
Hostinger
Digital Ocean
One.com
Melhore seu NETWORKING
https://digitalinnovation.one/
Participe de comunidades de desenvolvedores:
Fiquem a vontade para me adicionar ao linkedin.
E também para me seguir no https://github.com/toticavalcanti.
Código final da aula:
https://github.com/toticavalcanti
Quer aprender python3 de graça e com certificado? Acesse então:
https://workover.com.br/python-codigo-fluente
Canais do Youtube
Toti
Lofi Music Zone Beats
Backing Track / Play-Along
Código Fluente
Putz!
Vocal Techniques and Exercises
PIX para doações
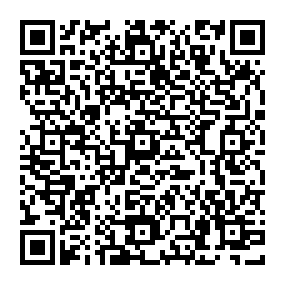
PIX Nubank
Aula 83 – Loja Online – Adicionando Campo Obrigatório
Introdução
Nesta aula, vamos dar continuidade ao nosso projeto de E-commerce utilizando o framework Django.
Se você acompanhou as aulas anteriores, já está familiarizado com os conceitos básicos do Django, como criação de projetos e aplicativos, modelos, formulários e administração.
Nesta aula em particular, iremos abordar a adição de campo obrigatório ao modelo de usuário.
Depois iremos desfazer essa obrigatoriedade do preenchimento do nome de usuário, deixando como opcional.
Vamos as mudanças para ter o campo full_name obrigatório!
django_ecommerce/e_commerce/accounts/models.py
from django.db import models
from django.contrib.auth.models import (
AbstractBaseUser, BaseUserManager
)
class UserManager(BaseUserManager):
def create_user(self, email, full_name, password = None, is_active = True, is_staff = False, is_admin = False):
if not email:
raise ValueError("O Usuário deve ter um endereço de email!")
if not password:
raise ValueError("O Usuário deve ter uma senha!")
if not full_name:
raise ValueError("O Usuário deve ter um nome completo!")
user_obj = self.model(
full_name = full_name,
email = self.normalize_email(email)
)
user_obj.set_password(password) # muda a senha
user_obj.staff = is_staff
user_obj.admin = is_admin
user_obj.active = is_active
user_obj.save(using=self._db)
return user_obj
def create_staffuser(self, email, full_name, password = None):
user = self.create_user(
email,
full_name,
password = password,
staff = True
)
return user
def create_superuser(self, email, full_name, password = None):
user = self.create_user(
email,
full_name,
password = password,
is_staff = True,
is_admin = True,
)
class User(AbstractBaseUser):
full_name = models.CharField(max_length=255, blank=True, null=True)
email = models.EmailField(max_length=255, unique=True)
active = models.BooleanField(default=True) # can do login
staff = models.BooleanField(default=False) # staff user, non superuser
admin = models.BooleanField(default=False) #superuser
timestamp = models.DateTimeField(auto_now_add=True)
# confirm = models.BooleanField(default=False)
# confirmed_date = models.DateTimeField(auto_now_add=True)
USERNAME_FIELD = 'email'
# USERNAME_FIELD and password are required by default
REQUIRED_FIELDS = ['full_name'] # ['full_name'] #python manage.py createsuperuser
objects = UserManager()
def __str__(self):
return self.email
def get_full_name(self):
return self.email
def get_short_name(self):
return self.email
def has_module_perms(self, app_label):
return True
def has_perm(self, perm, obj=None):
return True
@property
def is_staff(self):
return self.staff
@property
def is_admin(self):
return self.admin
@property
def is_active(self):
return self.active
class GuestEmail(models.Model):
email = models.EmailField()
active = models.BooleanField(default=True)
update = models.DateTimeField(auto_now=True)
timestamp = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.email
Coloque o full_name no fields do Forms
django_ecommerce/e_commerce/accounts/forms.py
from django import forms
from django.contrib.auth import get_user_model
from django.contrib.auth.forms import ReadOnlyPasswordHashField
User = get_user_model()
class UserAdminCreationForm(forms.ModelForm):
"""
A form for creating new users. Includes all the required
fields, plus a repeated password.
"""
password = forms.CharField(widget=forms.PasswordInput)
password_2 = forms.CharField(label='Confirm Password', widget=forms.PasswordInput)
class Meta:
model = User
fields = ['email']
def clean(self):
'''
Verify both passwords match.
'''
cleaned_data = super().clean()
password = cleaned_data.get("password")
password_2 = cleaned_data.get("password_2")
if password is not None and password != password_2:
self.add_error("password_2", "Your passwords must match")
return cleaned_data
def save(self, commit=True):
# Save the provided password in hashed format
user = super(UserAdminCreationForm, self).save(commit=False)
user.set_password(self.cleaned_data["password"])
if commit:
user.save()
return user
class UserAdminChangeForm(forms.ModelForm):
"""A form for updating users. Includes all the fields on
the user, but replaces the password field with admin's
password hash display field.
"""
password = ReadOnlyPasswordHashField()
class Meta:
model = User
fields = ['full_name', 'email', 'password', 'active', 'admin']
def clean_password(self):
# Regardless of what the user provides, return the initial value.
# This is done here, rather than on the field, because the
# field does not have access to the initial value
return self.initial["password"]
class GuestForm(forms.Form):
email = forms.EmailField()
class LoginForm(forms.Form):
username = forms.CharField()
password = forms.CharField(widget=forms.PasswordInput)
class RegisterForm(forms.Form):
username = forms.CharField()
email = forms.EmailField()
password = forms.CharField(widget=forms.PasswordInput)
password2 = forms.CharField(label='Confirm password', widget=forms.PasswordInput)
def clean_username(self):
username = self.cleaned_data.get('username')
qs = User.objects.filter(username=username)
if qs.exists():
raise forms.ValidationError("Esse usuário já existe, escolha outro nome.")
return username
def clean_email(self):
email = self.cleaned_data.get('email')
qs = User.objects.filter(email=email)
if qs.exists():
raise forms.ValidationError("Esse email já existe, tente outro!")
return email
def clean(self):
data = self.cleaned_data
password = self.cleaned_data.get('password')
password2 = self.cleaned_data.get('password2')
if password != password2:
raise forms.ValidationError("As senhas informadas devem ser iguais!")
return data
Agora vamos configurar a forma que queremos que apareça no form do user no painel admin, e colocar também no search_fields.
Admin
django_ecommerce/e_commerce/accounts/admin.py
from django.contrib import admin
from django.contrib.auth import get_user_model
from django.contrib.auth.models import Group
from django.contrib.auth.admin import UserAdmin as BaseUserAdmin
from .forms import UserAdminCreationForm, UserAdminChangeForm
from .models import GuestEmail
User = get_user_model()
class UserAdmin(BaseUserAdmin):
# The forms to add and change user instances
form = UserAdminChangeForm
add_form = UserAdminCreationForm
# The fields to be used in displaying the User model.
# These override the definitions on the base UserAdmin
# that reference specific fields on auth.User.
list_display = ['email', 'admin']
list_filter = ['admin', 'staff', 'active']
fieldsets = (
(None, {'fields': ('email', 'password')}),
('Personal info', {'fields': ('full_name',)}),
('Permissions', {'fields': ('admin', 'staff', 'active',)}),
)
# add_fieldsets is not a standard ModelAdmin attribute. UserAdmin
# overrides get_fieldsets to use this attribute when creating a user.
add_fieldsets = (
(None, {
'classes': ('wide',),
'fields': ('email', 'password', 'password_2')}
),
)
search_fields = ['email', 'full_name']
ordering = ['email']
filter_horizontal = ()
admin.site.register(User, UserAdmin)
# Remove Group Model from admin. We're not using it.
admin.site.unregister(Group)
class GuestEmailAdmin(admin.ModelAdmin):
search_fields = ['email']
class Meta:
model = GuestEmail
admin.site.register(GuestEmail, GuestEmailAdmin)
Como alteramos o modelo user, precisamos repercutir essas alterações no banco de dados, para isso, vamos fazer a migrations e migrate.
Migrations
python manage.py makemigrations
Migrate
python manage.py migrate
Agora, vamos criar um novo super usuário, para testar a mudança:
python manage.py createsuperuser
Primeiro tente criar sem informar um nome de usuário e veja o que acontece!
Viu? Não vai deixar criar o super usuário.
Colocamos o campo como obrigatório.
Mas, não é o que a gente quer, então vamos reverter isso.
Revertendo
django_ecommerce/e_commerce/accounts/models.py
from django.db import models
from django.contrib.auth.models import (
AbstractBaseUser, BaseUserManager
)
class UserManager(BaseUserManager):
def create_user(self, email, full_name = None, password = None, is_active = True, is_staff = False, is_admin = False):
if not email:
raise ValueError("O Usuário deve ter um endereço de email!")
if not password:
raise ValueError("O Usuário deve ter uma senha!")
user_obj = self.model(
full_name = full_name,
email = self.normalize_email(email)
)
user_obj.set_password(password) # muda a senha
user_obj.staff = is_staff
user_obj.admin = is_admin
user_obj.active = is_active
user_obj.save(using=self._db)
return user_obj
def create_staffuser(self, email, full_name = None, password = None):
user = self.create_user(
email,
full_name = full_name
password = password,
staff = True
)
return user
def create_superuser(self, email, full_name = None, password = None):
user = self.create_user(
email,
full_name = full_name
password = password,
is_staff = True,
is_admin = True,
)
class User(AbstractBaseUser):
full_name = models.CharField(max_length=255, blank=True, null=True)
email = models.EmailField(max_length=255, unique=True)
active = models.BooleanField(default=True) # can do login
staff = models.BooleanField(default=False) # staff user, non superuser
admin = models.BooleanField(default=False) #superuser
timestamp = models.DateTimeField(auto_now_add=True)
# confirm = models.BooleanField(default=False)
# confirmed_date = models.DateTimeField(auto_now_add=True)
USERNAME_FIELD = 'email'
# USERNAME_FIELD and password are required by default
REQUIRED_FIELDS = [] # ['full_name'] #python manage.py createsuperuser
objects = UserManager()
def __str__(self):
return self.email
def get_full_name(self):
if self.full_name:
return self.full_name
return self.email
def get_short_name(self):
return self.email
def has_module_perms(self, app_label):
return True
def has_perm(self, perm, obj=None):
return True
@property
def is_staff(self):
return self.staff
@property
def is_admin(self):
return self.admin
@property
def is_active(self):
return self.active
class GuestEmail(models.Model):
email = models.EmailField()
active = models.BooleanField(default=True)
update = models.DateTimeField(auto_now=True)
timestamp = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.email