Aula 10 – Tutorial Golang – Slices
Aula 10 – Tutorial Golang – Slices
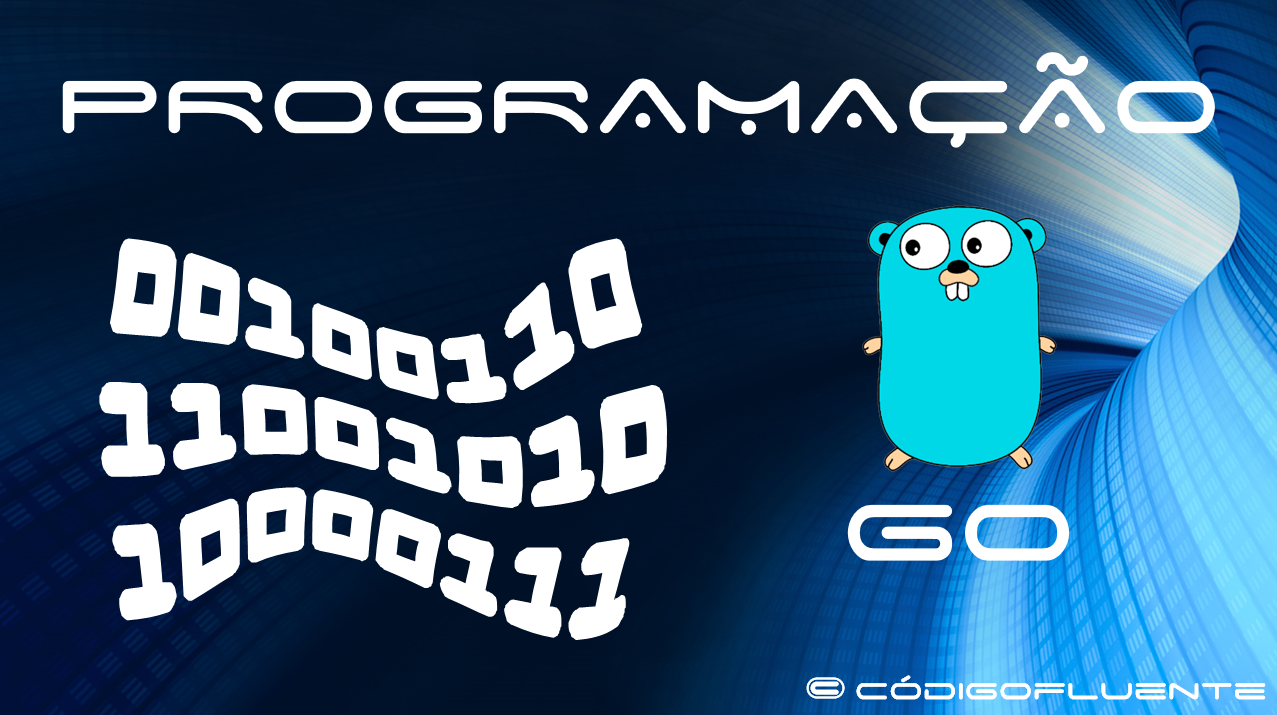
Tutorial Golang – Slices
Página principal do blog
Todas as aulas desse curso
Aula 09 Aula 11
Se gostarem do conteúdo dêem um joinha 👍 na página do Código Fluente no
Facebook
Esse é o link do código fluente no Pinterest
Meus links de afiliados:
Hostinger
Digital Ocean
One.com
Melhore seu NETWORKING
https://digitalinnovation.one/
Participe de comunidades de desenvolvedores:
Fiquem a vontade para me adicionar ao linkedin.
E também para me seguir no https://github.com/toticavalcanti.
Código final da aula:
https://github.com/toticavalcanti
Toti:
https://www.youtube.com/channel/UCUEtjLuDpcOvR3mIUr-viOA
Backing track / Play-along:
https://www.youtube.com/channel/UCT3TryVMqTqYBjf5g5WAHfA
Código Fluente
https://www.youtube.com/channel/UCgn-O-88XBAwdG9gUWkkb0w
Putz!
https://www.youtube.com/channel/UCZXop2-CECwyFYmHbhnAkAw
Aula 10 – Tutorial Golang – Slices
Slices
Slice é um tipo de dado chave em Go, fornecendo uma interface mais poderosa para sequências do que arrays.
Ao contrário dos arrays, os Slices são tipados apenas pelos elementos que contêm (não pelo número de elementos).
Para criar um Slice vazio com comprimento diferente de zero, use o comando make embutido.
Aqui, fazemos um slice de strings de comprimento 3 (inicialmente vazio).
s := make([]string, 3)
fmt.Println("set:", s)
Podemos definir e obter da mesma forma que os arrays.
s[0] = "a"
s[1] = "b"
s[2] = "c"
fmt.Println("set:", s)
fmt.Println("get:", s[2]))
A len() retorna o tamanho do Slice.
fmt.Println("len:", len(s))
Além dessas operações básicas, os Slices suportam várias outras que os tornam mais ricos do que os arrays.
Um é o método append, que retorna um Slice contendo um ou mais novos valores.
Observe que precisamos aceitar um valor de retorno de append, pois podemos obter um novo valor de Slice.
s = append(s, "d")
s = append(s, "e", "f")
fmt.Println("apd: ", s)
Os Slices também podem ser copiados.
Aqui, criamos um Slice c vazio, do mesmo tamanho de s e copiamos o conteúdo de s para c.
c := make([]string, len(s))
copy(c, s)
fmt.Println("cpy:", c)
Os Slices oferecem suporte a um operador “fatia – slice” com a sintaxe [baixo: alto].
Por exemplo, para obter uma fatia com os elementos s[2], s[3] e s[4].
l := s[2:5]
fmt.Println("sl1:", l))
Isso fatia até s[4] excluindo s[5].
l = s[:5]
fmt.Println("sl2:", l)
E isso fatia do s[2] até o fim.
l = s[2:]
fmt.Println("sl3:", l)
Podemos declarar e inicializar um slice em uma única linha também.
t := []string{"g", "h", "i"}
fmt.Println("dcl:", t)
Os Slices podem ser compostos em estruturas de dados multidimensionais.
O comprimento dos Slices internos pode variar, ao contrário de matrizes multidimensionais.
twoD := make([][]int, 3)
for i := 0; i < 3; i++ {
innerLen := i + 1
twoD[i] = make([]int, innerLen)
for j := 0; j < innerLen; j++ {
twoD[i][j] = i + j
}
}
fmt.Println("2d: ", twoD)
Observe que, embora os slices sejam de tipos diferentes dos arrays, eles são renderizados de maneira semelhante por fmt.Println().
Código Completo
// _Slices_ are a key data type in Go, giving a more
// powerful interface to sequences than arrays.
package main
import "fmt"
func main() {
// Unlike arrays, slices are typed only by the
// elements they contain (not the number of elements).
// To create an empty slice with non-zero length, use
// the builtin `make`. Here we make a slice of
// `string`s of length `3` (initially zero-valued).
s := make([]string, 3)
fmt.Println("emp:", s)
// We can set and get just like with arrays.
s[0] = "a"
s[1] = "b"
s[2] = "c"
fmt.Println("set:", s)
fmt.Println("get:", s[2])
// `len` returns the length of the slice as expected.
fmt.Println("len:", len(s))
// In addition to these basic operations, slices
// support several more that make them richer than
// arrays. One is the builtin `append`, which
// returns a slice containing one or more new values.
// Note that we need to accept a return value from
// `append` as we may get a new slice value.
s = append(s, "d")
s = append(s, "e", "f")
fmt.Println("apd:", s)
// Slices can also be `copy`'d. Here we create an
// empty slice `c` of the same length as `s` and copy
// into `c` from `s`.
c := make([]string, len(s))
copy(c, s)
fmt.Println("cpy:", c)
// Slices support a "slice" operator with the syntax
// `slice[low:high]`. For example, this gets a slice
// of the elements `s[2]`, `s[3]`, and `s[4]`.
l := s[2:5]
fmt.Println("sl1:", l)
// This slices up to (but excluding) `s[5]`.
l = s[:5]
fmt.Println("sl2:", l)
// And this slices up from (and including) `s[2]`.
l = s[2:]
fmt.Println("sl3:", l)
// We can declare and initialize a variable for slice
// in a single line as well.
t := []string{"g", "h", "i"}
fmt.Println("dcl:", t)
// Slices can be composed into multi-dimensional data
// structures. The length of the inner slices can
// vary, unlike with multi-dimensional arrays.
twoD := make([][]int, 3)
for i := 0; i < 3; i++ {
innerLen := i + 1
twoD[i] = make([]int, innerLen)
for j := 0; j < innerLen; j++ {
twoD[i][j] = i + j
}
}
fmt.Println("2d: ", twoD)
}
E pra executar é só entrar na pasta onde tá o arquivo switch.go e digitar:
go run slices.go